Published
- 3 min read
Angular Facts 1
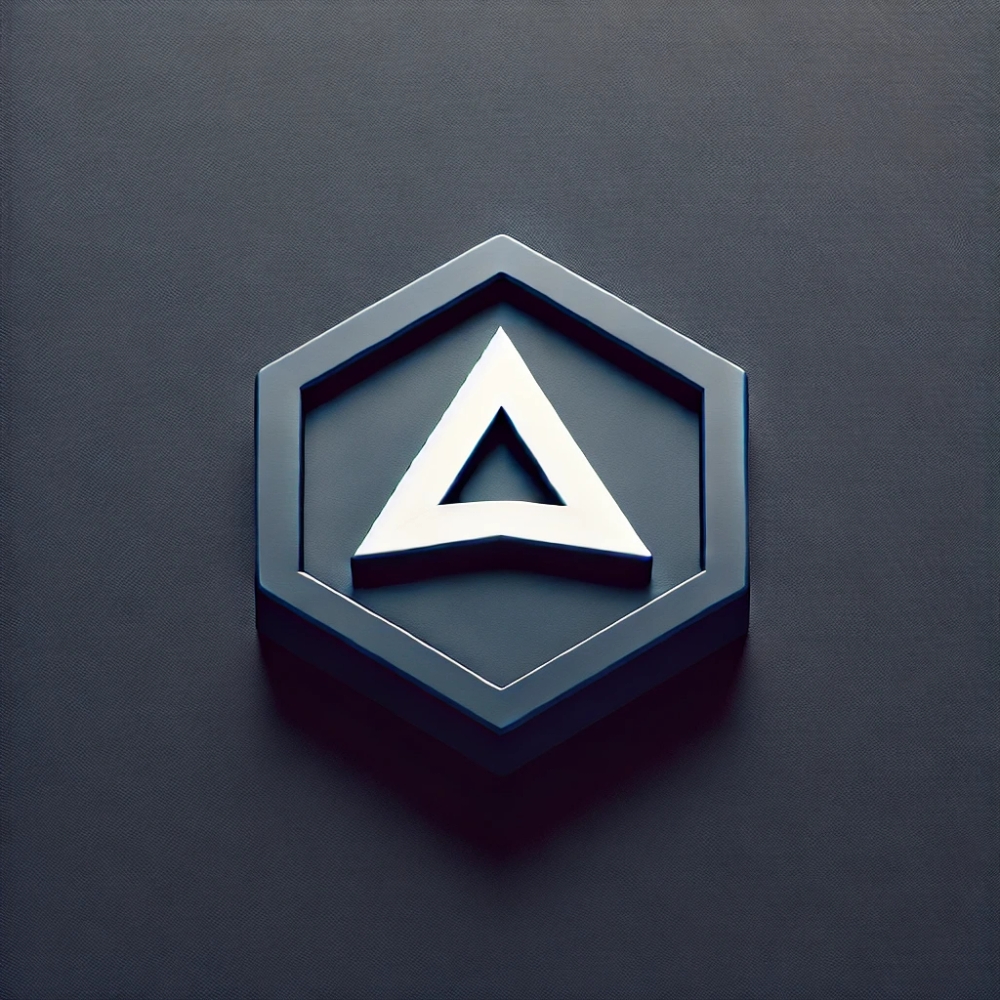
bootstrapApplication
- The
bootstrapApplication
function is used to bootstrap an Angular application. - It is a function that is exported from the
@angular/platform-browser
module. - It is used to bootstrap the root component of the application.
import { bootstrapApplication } from '@angular/platform-browser';
// The below code will bootstrap the root component of the application.
bootstrapApplication(AppComponent);
ApplicationConfig
- The
ApplicationConfig
interface is used to define the configuration object for thebootstrapApplication
function. - It is an interface that is exported from the
@angular/core
module. - It is used to define the configuration object for the
bootstrapApplication
function.
import { ApplicationConfig } from '@angular/core';
// The below code will define the configuration object for the bootstrapApplication function.
export const appConfig: ApplicationConfig = {
providers: []
};
bootstrapApplication(AppComponent, appConfig);
provideZoneChangeDetection
- The
provideZoneChangeDetection
function is used to provide the zone change detection strategy. - It is a function that is exported from the
@angular/core
module.
import { provideZoneChangeDetection } from '@angular/core';
// The below code will provide the zone change detection strategy with bootstrapApplication
bootstrapApplication(AppComponent, {
providers: [provideZoneChangeDetection()]
});
provideRouter
- The
provideRouter
function is used to provide the router configuration. - It is a function that is exported from the
@angular/router
module.
import { provideRouter } from '@angular/router';
// The below code will provide the router configuration with bootstrapApplication
bootstrapApplication(AppComponent, {
providers: [provideRouter(routes)]
});
Routes
- The
Routes
interface is used to define the routes configuration for the router. - It is an interface that is exported from the
@angular/router
module. - The
loadChildren
property is used to load a module lazily. - The
path
property is used to define the path for the route. - The
component
property is used to define the component for the route. - The
children
property is used to define child routes. - The
redirectTo
property is used to redirect to another route. - The
pathMatch
property is used to define the matching strategy for the route. - The
data
property is used to pass additional data to the route.
import { Routes } from '@angular/router';
// The below code will define the routes configuration for the router
const routes: Routes = [ ]
// Adding a route containing path and component
routes.push({
path: 'hello',
component: HelloComponent
});
// Adding a route with loadChildren
routes.push({
path: 'admin',
loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule)
});
// Adding a route with children
routes.push({
path: 'dashboard',
children: [
{ path: 'overview', component: DashboardOverviewComponent },
{ path: 'settings', component: DashboardSettingsComponent }
]
});
// Adding a route with redirectTo
routes.push({
path: '',
redirectTo: '/dashboard',
pathMatch: 'full'
});
// Adding a route with pathMatch
routes.push({
path: 'details',
component: DetailsComponent,
pathMatch: 'full'
});
// Adding a route with data
routes.push({
path: 'example',
pathComponent: ExampleComponent,
data: { title: 'Example Page' }
});