Published
- 7 min read
Flutter Facts 1
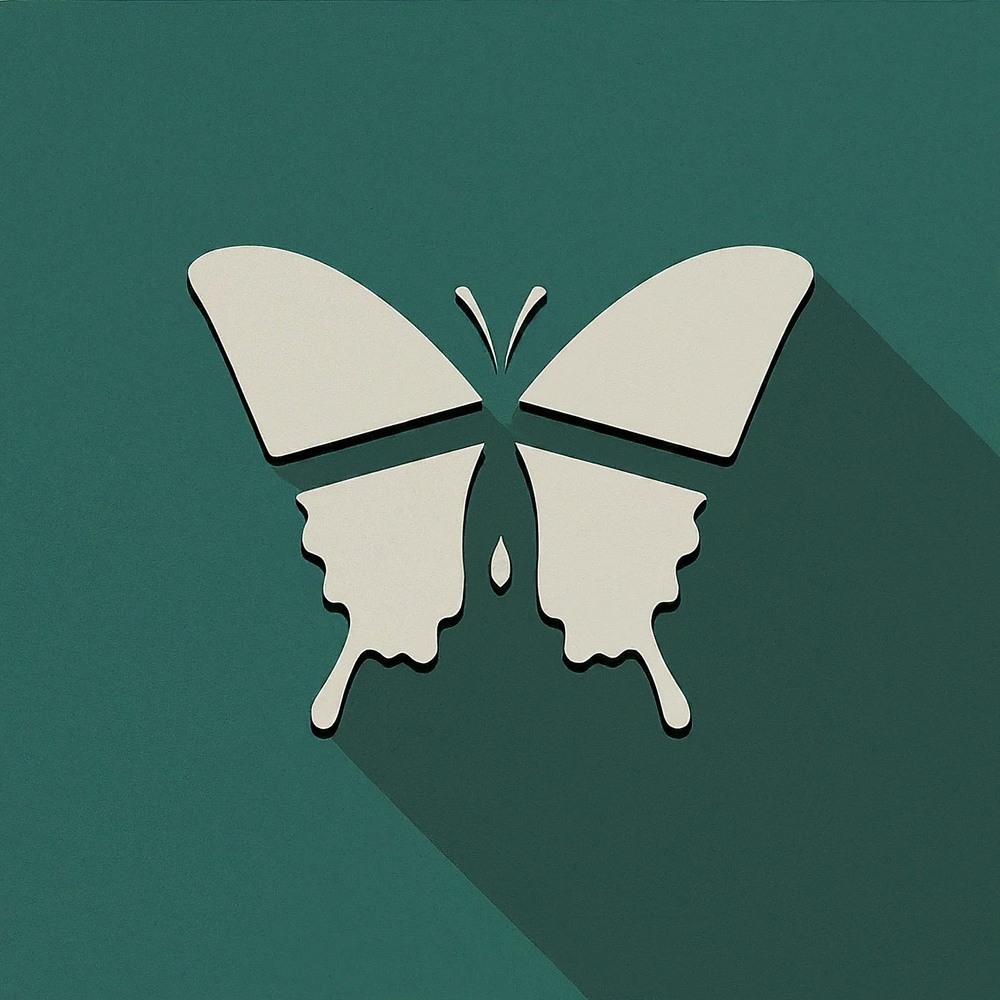
Flutter State Management
- State management is a crucial aspect of building applications in Flutter.
- State management refers to the management of the data that is used by the application.
- In Flutter, there are various ways to manage the state of an application.
- Some of the popular state management solutions in Flutter include
setState
,Provider
,Bloc
,GetX
,Riverpod
, andMobX
. - The choice of state management solution depends on the complexity of the application, the team’s familiarity with the solution, and the performance requirements.
setState
is the simplest way to manage the state in Flutter and is suitable for small applications with minimal state.Provider
is a popular state management solution that uses theInheritedWidget
to provide the data to the widgets in the widget tree.Bloc
is a state management solution that uses theBLoC
pattern to separate the business logic from the UI.GetX
is a lightweight state management solution that provides reactive state management and dependency injection.Riverpod
is a provider package that offers a simple and powerful way to manage the state in Flutter applications.MobX
is a state management solution that uses theObservable
pattern to manage the state in Flutter applications.
// The below code snippet shows an example of using the Provider package for state management.
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Provider Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'Counter Value:',
),
Consumer<Counter>(
builder: (context, counter, child) {
return Text(
'${counter.count}',
style: Theme.of(context).textTheme.headline4,
);
},
),
ElevatedButton(
onPressed: () {
Provider.of<Counter>(context, listen: false).increment();
},
child: Text('Increment'),
),
],
),
),
),
);
}
}
Flutter Widgets
- Widgets are the building blocks of a Flutter application.
- Widgets are used to create the user interface of the application.
- Flutter provides a rich set of widgets that can be used to build complex and beautiful user interfaces.
- Widgets can be categorized into two main types: StatelessWidget and StatefulWidget.
- StatelessWidget is a widget that does not have any internal state and is immutable.
- StatefulWidget is a widget that has internal state and can change over time.
- Some of the commonly used widgets in Flutter include
Container
,Row
,Column
,Text
,Image
,ListView
,GridView
,AppBar
,Scaffold
,TextField
,Button
,AlertDialog
,BottomNavigationBar
, andTabBar
.
// The below code snippet shows an example of using the Container widget in Flutter.
Container(
color: Colors.blue,
width: 100,
height: 100,
child: Center(
child: Text(
'Hello, Flutter!',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
),
)
// The below code snippet shows an example of using the Row and Column widgets in Flutter.
Row(
children: <Widget>[
Icon(Icons.star),
Icon(Icons.star),
Icon(Icons.star),
],
)
Column(
children: <Widget>[
Icon(Icons.star),
Icon(Icons.star),
Icon(Icons.star),
],
)
// The below code snippet shows an example of using the Text widget in Flutter.
Text(
'Hello, Flutter!',
style: TextStyle(
color: Colors.blue,
fontSize: 20,
),
)
// The below code snippet shows an example of using the ListView widget in Flutter.
ListView(
children: <Widget>[
ListTile(
title: Text('Item 1'),
),
ListTile(
title: Text('Item 2'),
),
ListTile(
title: Text('Item 3'),
),
],
)
// The below code snippet shows an example of using the GridView widget in Flutter.
GridView.count(
crossAxisCount: 2,
children: <Widget>[
Container(
color: Colors.blue,
child: Center(
child: Text('Item 1'),
),
),
Container(
color: Colors.green,
child: Center(
child: Text('Item 2'),
),
),
Container(
color: Colors.red,
child: Center(
child: Text('Item 3'),
),
),
],
)
// The below code snippet shows an example of using the AppBar and Scaffold widgets in Flutter.
AppBar(
title: Text('My App'),
)
Scaffold(
appBar: AppBar(
title: Text('My App'),
),
body: Center(
child: Text('Hello, Flutter!'),
),
)
// The below code snippet shows an example of using the TextField widget in Flutter.
TextField(
decoration: InputDecoration(
hintText: 'Enter your name',
),
)
// The below code snippet shows an example of using the Button widget in Flutter.
ElevatedButton(
onPressed: () {
// Add your action here
},
child: Text('Click Me'),
)
// The below code snippet shows an example of using the AlertDialog widget in Flutter.
ElevatedButton(
onPressed: () {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Text('Alert'),
content: Text('This is an alert dialog.'),
actions: <Widget>[
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('OK'),
),
],
);
},
);
},
child: Text('Show Alert'),
)
Flutter Navigation
- Navigation is an essential part of building mobile applications in Flutter.
- Navigation refers to moving between different screens or pages in the application.
- Flutter provides various navigation options to navigate between screens, such as
Navigator
,MaterialPageRoute
,Named routes
, andBottomNavigationBar
. - The
Navigator
class is used to manage the stack of routes in the application. - The
MaterialPageRoute
class is used to define a route that transitions to a new screen using a material design animation. - Named routes are used to define routes with a unique name that can be accessed from anywhere in the application.
- The
BottomNavigationBar
widget is used to create a bottom navigation bar with multiple tabs for navigating between screens. - Navigation in Flutter can be done using the
Navigator.push
method to push a new route onto the stack,Navigator.pop
method to remove the current route from the stack, andNavigator.pushNamed
method to navigate to a named route.
// The below code snippet shows an example of using the Navigator class for navigation in Flutter.
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(),
),
);
// The below code snippet shows an example of using named routes for navigation in Flutter.
MaterialApp(
initialRoute: '/',
routes: {
'/': (context) => FirstScreen(),
'/second': (context) => SecondScreen(),
},
);
// The below code snippet shows an example of using the BottomNavigationBar widget for navigation in Flutter.
BottomNavigationBar(
items: [
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.search),
label: 'Search',
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
label: 'Settings',
),
],
currentIndex: _selectedIndex,
onTap: _onItemTapped,
)
Flutter Packages
- Flutter packages are reusable libraries that provide additional functionality to Flutter applications.
- Flutter packages can be used to add features such as animations, networking, state management, and more to your application.
- Flutter packages are published on pub.dev, the official package repository for Flutter.
- To use a package in your Flutter application, you need to add it to the
pubspec.yaml
file under thedependencies
section. - Some popular Flutter packages include
http
for making HTTP requests,shared_preferences
for storing key-value pairs locally,provider
for state management,firebase_core
for integrating Firebase services, andflutter_svg
for working with SVG images. - You can search for Flutter packages on pub.dev and find packages that suit your needs.
// The below code snippet shows an example of adding a package to the pubspec.yaml file.
dependencies:
http: ^0.13.3
// The below code snippet shows an example of using the http package to make an HTTP request in Flutter.
import 'package:http/http.dart' as http;
void fetchData() async {
var response = await http.get(Uri.parse('https://api.example.com/data'));
if (response.statusCode == 200) {
print(response.body);
} else {
print('Failed to fetch data');
}
}
Flutter Plugins
- Flutter plugins are packages that provide access to platform-specific APIs and services in Flutter applications.
- Flutter plugins allow you to interact with device features such as the camera, location, sensors, and more.
- Flutter plugins are published on pub.dev and can be added to your Flutter application like any other package.
- Some popular Flutter plugins include
camera
for accessing the device camera,geolocator
for getting the device’s location,connectivity
for checking network connectivity, andfirebase_auth
for integrating Firebase authentication services. - Flutter plugins provide a bridge between the Flutter framework and the native platform code, allowing you to access native features from your Flutter application.
- You can search for Flutter plugins on pub.dev and find plugins that provide the functionality you need.
// The below code snippet shows an example of adding a plugin to the pubspec.yaml file.
dependencies:
camera: ^0.9.4
// The below code snippet shows an example of using the camera plugin to access the device camera in Flutter.
import 'package:camera/camera.dart';
void initCamera() async {
final cameras = await availableCameras();
final firstCamera = cameras.first;
runApp(
MaterialApp(
home: CameraApp(camera: firstCamera),
),
);
}