Published
- 5 min read
UI Facts 1
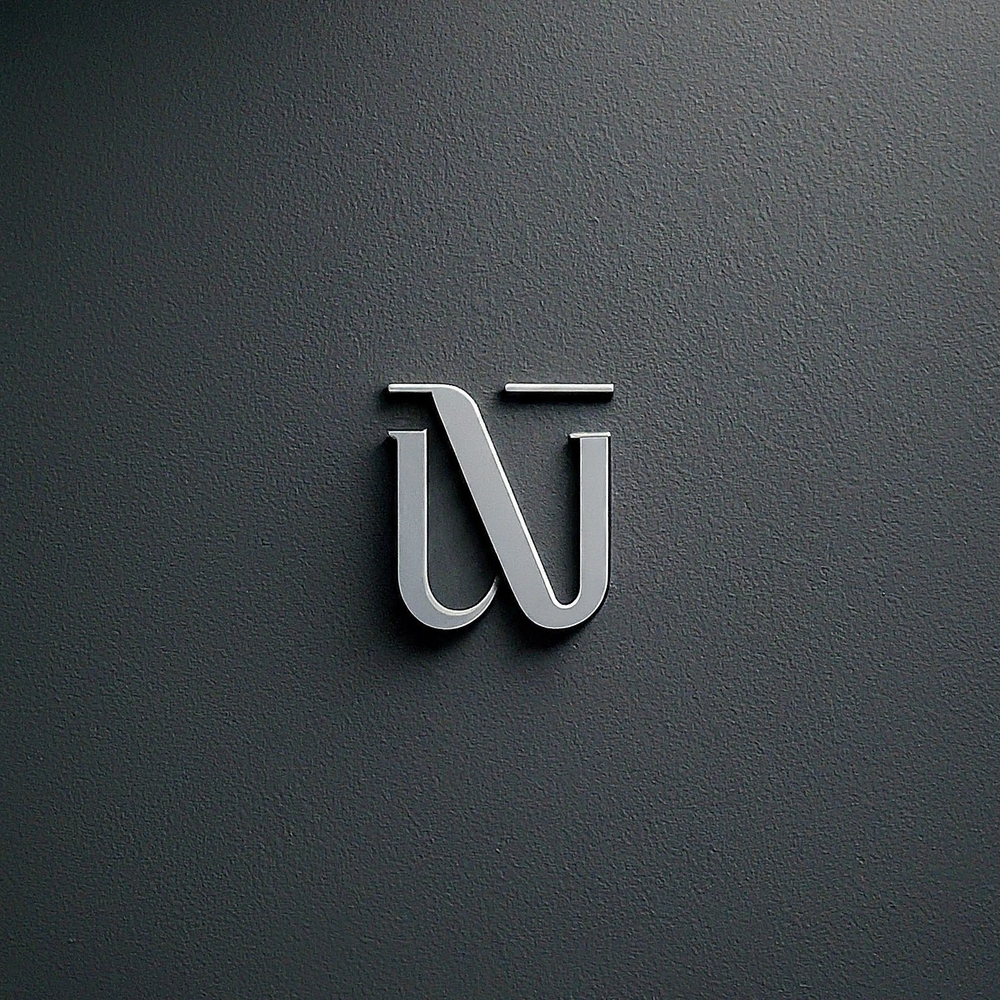
Navbar
- The
Navbar
is a UI component that is used to provide navigation links to the user. - It is a horizontal bar that contains links to different sections of a website.
- The
Navbar
is usually placed at the top of the page. - It can contain links to different pages, dropdown menus, search bars, and other interactive elements.
- The
Navbar
can be styled using CSS to match the design of the website. - The
Navbar
can be made responsive using media queries to adapt to different screen sizes. - The
Navbar
can be fixed at the top of the page to provide easy access to navigation links. - The
Navbar
can be customized with different colors, fonts, icons, and animations to enhance the user experience.
// The below code will create a basic Navbar component.
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
// The below html and css code will create a responsive Navbar component
<style>
nav {
display: flex;
justify-content: space-between;
align-items: center;
padding: 1rem;
background-color: #333;
color: white;
}
ul {
display: flex;
list-style: none;
}
li {
margin-right: 1rem;
}
a {
color: white;
text-decoration: none;
}
@media (max-width: 768px) {
ul {
display: none;
}
.menu-icon {
display: block;
cursor: pointer;
}
.menu {
display: none;
flex-direction: column;
position: absolute;
top: 100%;
left: 0;
background-color: #333;
width: 100%;
}
.menu-toggle:checked + .menu {
display: flex;
}
}
</style>
<nav>
<div class="logo">Logo</div>
<input type="checkbox" id="menu-toggle">
<label for="menu-toggle" class="menu-icon">☰</label>
<ul class="menu">
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
// The below html and css code will create a fixed Navbar component.
<style>
.fixed-navbar {
position: fixed;
top: 0;
left: 0;
width: 100%;
background-color: #333;
color: white;
padding: 1rem;
}
.fixed-navbar ul {
display: flex;
list-style: none;
}
.fixed-navbar li {
margin-right: 1rem;
}
.fixed-navbar a {
color: white;
text-decoration: none;
}
</style>
<nav class="fixed-navbar">
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
flex
- The
flex
property in CSS is used to create flexible layouts. - It is a shorthand property for setting the
flex-grow
,flex-shrink
, andflex-basis
properties. - The
flex-grow
property specifies how much a flex item should grow relative to the other flex items. - The
flex-shrink
property specifies how much a flex item should shrink relative to the other flex items. - The
flex-basis
property specifies the initial size of a flex item before any remaining space is distributed. - The
flex
property can be used to set all three properties at once in the following order:flex-grow
,flex-shrink
,flex-basis
. - The
flex
property can be used with theflex-direction
,justify-content
, andalign-items
properties to create different layouts. - The
flex
property can be used with media queries to create responsive layouts that adapt to different screen sizes. - The
flex
property is widely used in modern web design to create flexible and responsive layouts.
// The below code will create a flex container with three flex items.
<style>
.flex-container {
display: flex;
justify-content: space-between;
align-items: center;
}
.flex-item {
flex: 1 1 30%;
padding: 1rem;
margin: 0.5rem;
background-color: #f0f0f0;
}
</style>
<div class="flex-container">
<div class="flex-item">Item 1</div>
<div class="flex-item">Item 2</div>
<div class="flex-item">Item 3</div>
</div>
// The below code will create a responsive flex container with two flex items.
<style>
.responsive-flex-container {
display: flex;
flex-direction: column;
}
.responsive-flex-item {
flex: 1 1 50%;
padding: 1rem;
margin: 0.5rem;
background-color: #f0f0f0;
}
@media (min-width: 768px) {
.responsive-flex-container {
flex-direction: row;
}
}
</style>
<div class="responsive-flex-container">
<div class="responsive-flex-item">Item 1</div>
<div class="responsive-flex-item">Item 2</div>
</div>
Modal
- The
Modal
is a UI component that is used to display content on top of the main content. - It is a popup window that appears in the center of the screen and overlays the main content.
- The
Modal
is used to show important information, alerts, notifications, or interactive elements to the user. - The
Modal
can contain text, images, videos, forms, buttons, and other interactive elements. - The
Modal
can be styled using CSS to match the design of the website. - The
Modal
can be opened and closed using JavaScript or CSS animations. - The
Modal
can be made responsive using media queries to adapt to different screen sizes. - The
Modal
can be customized with different colors, fonts, icons, and animations to enhance the user experience.
// The below code will create a basic Modal component.
<style>
.modal {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
z-index: 999;
}
.modal-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 2rem;
background-color: white;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.5);
}
.close {
position: absolute;
top: 1rem;
right: 1rem;
cursor: pointer;
}
</style>
<div class="modal" id="modal">
<div class="modal-content">
<span class="close" onclick="closeModal()">×</span>
<h2>Modal Title</h2>
<p>Modal Content</p>
</div>
</div>
<button onclick="openModal()">Open Modal</button>
<script>
function openModal() {
document.getElementById('modal').style.display = 'block';
}
function closeModal() {
document.getElementById('modal').style.display = 'none';
}
</script>
// The below code will create a responsive Modal component.
<style>
@media (max-width: 768px) {
.modal-content {
width: 90%;
}
}
</style>
<div class="modal" id="modal">
<div class="modal-content">
<span class="close" onclick="closeModal()">×</span>
<h2>Modal Title</h2>
<p>Modal Content</p>
</div>
</div>